About User Data
Updated: 01/26/2023
In Cubism 3.1 or later, a “user data” function is available to add string information to an ArtMesh.
The string data shown in the .userdata3.json file can be retrieved programmatically as information associated with the ArtMesh and can be used as a discriminative material for various processing tasks on the ArtMesh.
As an example, you can use it as follows.
- The character can be customized by adding tags such as “Skin” and “Hair” and changing the color of the rendering depending on the tag.
- Describe parameters such as manipulating transparency, and manipulate parameters by the distance from the average coordinates of the mesh to light sources, etc., to express illumination.
- Put particles only on the tagged mesh.
Create an Instance
User data is described in the .userdata3.json file and handled by the CubismModelUserData class.
Loading is done with one of the following functions:
- CubismModelUserData::Create function in Native (C++)
- CubismModelUserData.create function in Web (TypeScript)
- CubismModelUserData.create function in Java
// C++ csmString path = _modelSetting->GetUserDataFile(); path = _modelHomeDir + path; buffer = CreateBuffer(path.GetRawString(), &size); CubismModelUserData* _modelUserData = CubismModelUserData::Create(buffer, size); DeleteBuffer(buffer, path.GetRawString());
// TypeScript let path: string = _modelSetting.getUserDataFile(); path = _modelHomeDir + path; fetch(path).then( (response) => { return response.arrayBuffer(); } ).then( (arrayBuffer) => { let buffer: ArrayBuffer = arrayBuffer; let size: number = buffer.byteLength; let _modelUserData: CubismModelUserData = CubismModelUserData.create(buffer, size); deleteBuffer(buffer, path); } );
// Java String path = modelSetting.getUserDataFile(); path = modelHomeDir + path; buffer = createBuffer(path); CubismModelUserData modelUserData = CubismModelUserData.create(buffer);
Access
User data can be accessed using one of the following functions.
- CubismModelUserData::GetArtMeshUserDatas function in Native (C++)
- CubismModelUserData.getArtMeshUserDatas function in Web (TypeScript)
- CubismModelUserData.getArtMeshUserData function in Java
A dynamic array containing the CubismModelUserDataNode structure is returned, which is accessed like a normal array.
For Java, since a List containing the CubismModelUserDataNode class is returned, access it like a normal List.
// C++ const csmVector<const CubismModelUserData::CubismModelUserDataNode*>& ans = _modelUserData->GetArtMeshUserDatas(); for ( csmUint32 i = 0; i < ans.GetSize(); ++i) { CubismIdHandle handle = ans[i]->TargetId; }
// TypeScript const ans: csmVector<CubismModelUserData.CubismModelUserDataNode> = _modelUserData.getArtMeshUserDatas(); for(let i: number = 0; i < ans.getSize(); ++i) { let handle: CubismIdHandle = ans[i].targetId; }
// Java final List<CubismModelUserDataNode> ans = modelUserData.getArtMeshUserData(); for(int i = 0; i < ans.size(); i++){ CubismId handle = ans.get(i).targetId; }
Discard
The CubismModelUserData class must also be destroyed at the time the model is released.
// C++ CubismModelUserData::Delete(_modelUserData);
// TypeScript CubismModelUserData.delete(_modelUserData);
Java does not need to be disposed because garbage collection is responsible for deallocation.
Display a Range of Userdata
In the Live2D Cubism SDK for Native Cocos2d-x sample, by setting DebugDrawRectEnable in LAppDefine.cpp to true, the position where the HitArea and Userdata ArtMeshes are located will be displayed as a rectangle.
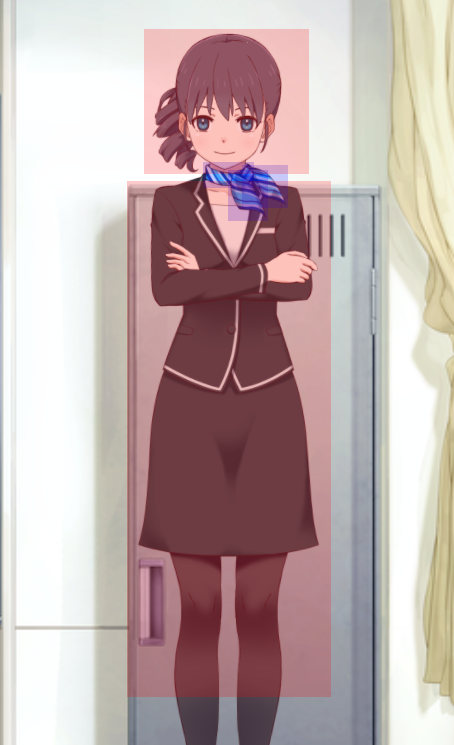
Red rectangles are the HitArea and the blue rectangles on the neck scarf are the area where the Userdata exists.