[Web] Multiply Color/Screen Color
Updated: 05/15/2025
By applying Multiply Color and Screen Color to the model, tints can be changed in real time.
Multiply Color and Screen Color set in Cubism Editor are applied by using Cubism Web Framework after Cubism 4.2 without any additional coding.
See the “Multiply Color/Screen Color” page in the Editor manual for details on setting the Multiply Color and Screen Color in the Cubism Editor.
Also, by coding as needed, it is possible to manipulate Multiply Color and Screen Color from the SDK and perform the following operations.
- Apply Multiply Color and Screen Color interactively.
- Apply Multiply Color and Screen Color settings that have not been set in the Cubism Editor.
- Disable Multiply Color and Screen Color set in the Cubism Editor.
The following is an explanation of the procedure.
Procedures
The process is as follows.
- Overwrite flag settings for Multiply Color and Screen Color
- Multiply Color and Screen Color settings
- Model drawing
Overwrite flag settings for Multiply Color and Screen Color
First, set the overwrite flag for Multiply Color and Screen Color to true. The default is false.
model.getModel().setOverrideFlagForModelMultiplyColors(true); // Multiply color overwrite flag model.getModel().setOverrideFlagForModelScreenColors(true); // Screen color overwrite flag
public setOverrideFlagForModelMultiplyColors(value: boolean) and public setOverrideFlagForModelScreenColors(value: boolean) are defined in the Framework’s CubismModel class.
The Cubism SDK for Web sample project defines the LAppModel class with CubismModel as the base class to manipulate models, and the model in the above code is the LAppModel class.
getModel() is used as an intermediary to call functions of the CubismModel class from the LAppModel class.
Multiply Color and Screen Color settings
Define Multiply Color and Screen Color and set them to the model.
The code below shows the set values for setting the Multiply Color to red and the Screen Color to green for all Drawables.
RGBA can be used for the setting color.
for (let i = 0; i < model.getModel().getDrawableCount(); i++) { let multiplyColor: CubismTextureColor = new CubismTextureColor(); // Multiply color multiplyColor.R = 1.0; multiplyColor.G = 0.5; multiplyColor.B = 0.5; multiplyColor.A = 1.0; let screenColor: CubismTextureColor = new CubismTextureColor(); // Screen color screenColor.R = 0.0; screenColor.G = 0.5; screenColor.B = 0.0; screenColor.A = 1.0; model.getModel().setMultiplyColor(i, multiplyColor); model.getModel().setScreenColor(i, screenColor); }
Before applying Multiply Color and Screen Color
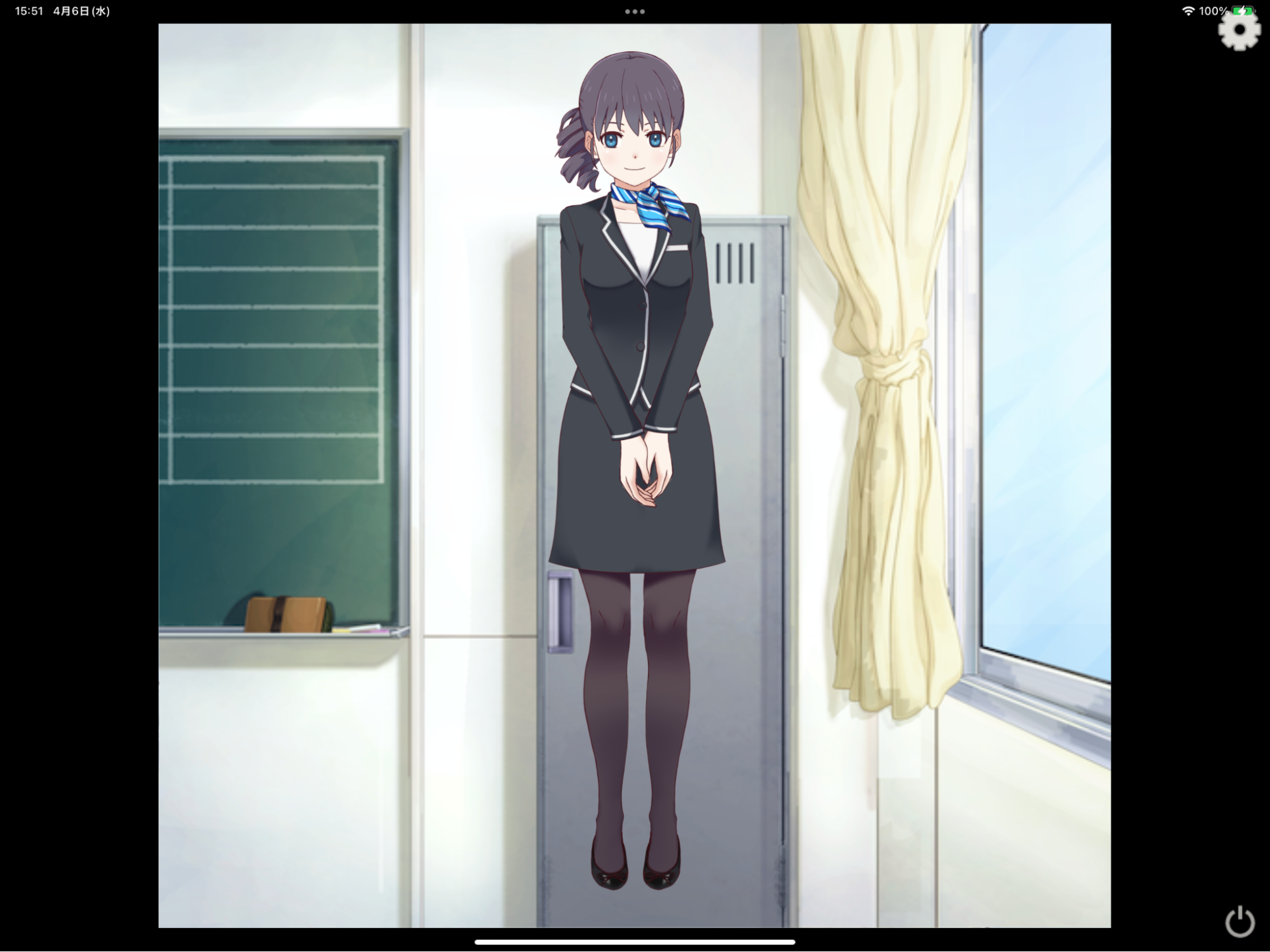
After applying red to Multiply Color and green to Screen Color
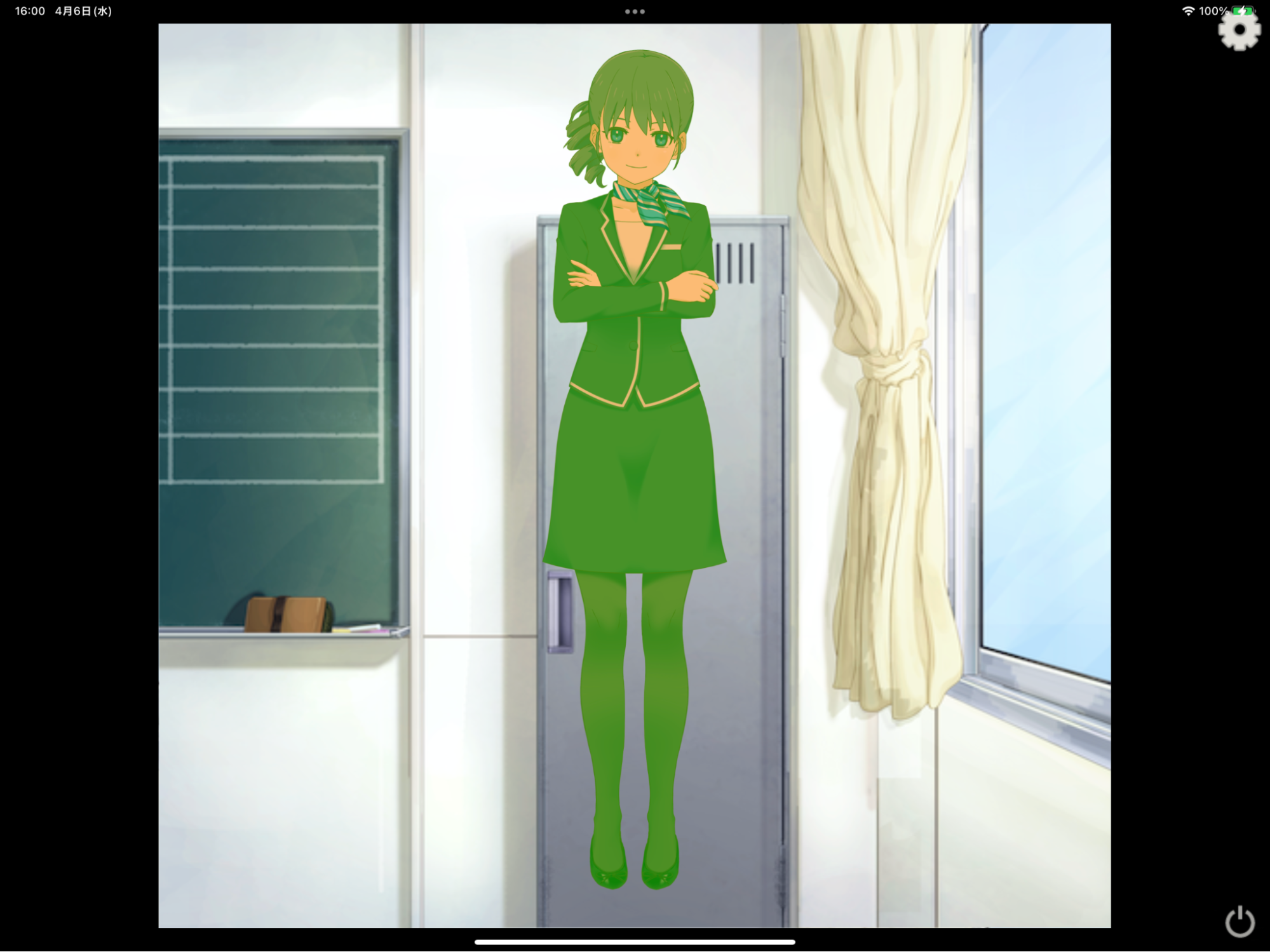
First of all, for the loop process performed by the DrawableCount of the model, the Multiply Color and Screen Color processing is performed by all Drawables every frame.
Tips
In this case, the same Multiply Color and Screen Color are set for all Drawables, but it is possible to set different Multiply Color and Screen Color for each Drawable index.
The argument i is the index of the Drawable.
Tips
To disable the Multiply Color and Screen Color set in the Cubism Editor, set 0 for the Multiply Color and 1 for the Screen Color. This will be the initial value of each.
let multiplyColor: CubismTextureColor = new CubismTextureColor(); // Multiply color multiplyColor.R = 1.0; multiplyColor.G = 1.0; multiplyColor.B = 1.0; multiplyColor.A = 1.0; let screenColor: CubismTextureColor = new CubismTextureColor(); // Screen color screenColor.R = 0.0; screenColor.G = 0.0; screenColor.B = 0.0; screenColor.A = 1.0;
Model drawing
model.draw(projection);
By calling the model’s draw() function, the model is drawn including Multiply Color and Screen Color by the internal rendering process of Cubism Web Framework.
This function is also called in the Cubism SDK for Web sample project.
Other Related Functions
Obtain overwrite flag for Multiply Color and Screen Color
If the return value is true, the color information set by the SDK is given priority. If the return value is false, the color information of the model is given priority.
public getOverrideFlagForModelMultiplyColors(): boolean // Get multiply color overwrite flag. public getOverrideFlagForModelScreenColors(): boolean // Get screen color overwrite flag.
Multiply Color and Screen Color settings
The above describes the setting method with cubismrenderer.CubismTextureColoras an argument, but there is also a function that can set RGBA directly. (Input value 0.0–1.0)
public setMultiplyColorByRGBA(index: number, r: number, g: number, b: number, a = 1.0) // Set multiply color public setScreenColorByRGBA(index: number, r: number, g: number, b: number, a = 1.0) // Set screen color
Obtain Multiply Color and Screen Color
Get the Multiply Color and Screen Color of each Drawable with the CubismTextureColor type.
public getMultiplyColor(index: number): CubismTextureColor public getScreenColor(index: number): CubismTextureColor
The argument indexis the index of the Drawable.
Internal Processing of Cubism Web Framework
This is the Framework’s internal process for representing Multiply Color and Screen Color.
The process is as follows.
- Get Multiply Color and Screen Color of the model from Cubism Core
- Determine the acquired Multiply Color and Screen Color by the overwrite flag.
- Set the acquired Multiply Color and Screen Color to the shader program.
- Add Multiply Color and Screen Color when calculating texture color in the fragment shader.
Tips
Get the model’s Multiply Color and Screen Color from Cubism Core in RGBA, but A (transparency) is not set in the Cubism Editor.
Get Multiply Color and Screen Color of the model from Cubism Core
Get Multiply Color and Screen Color from the model for all Drawables using Cubism Core’s API.
public getDrawableMultiplyColor(drawableIndex: number): CubismTextureColor { const multiplyColors: Float32Array = this._model.drawables.multiplyColors; const index = drawableIndex * 4; const multiplyColor: CubismTextureColor = new CubismTextureColor(); multiplyColor.R = multiplyColors[index]; multiplyColor.G = multiplyColors[index + 1]; multiplyColor.B = multiplyColors[index + 2]; multiplyColor.A = multiplyColors[index + 3]; return multiplyColor; } public getDrawableScreenColor(drawableIndex: number): CubismTextureColor { const screenColors: Float32Array = this._model.drawables.screenColors; const index = drawableIndex * 4; const screenColor: CubismTextureColor = new CubismTextureColor(); screenColor.R = screenColors[index]; screenColor.G = screenColors[index + 1]; screenColor.B = screenColors[index + 2]; screenColor.A = screenColors[index + 3]; return screenColor; }
Core’s API is as follows.
multiplyColors: Float32Array; // Get multiply color screenColors: Float32Array; // Get screen color
At initialization of the CubismModel class, all Drawables are retrieved and held in a member array.
To set multiply and screen colors on the SDK side, set setOverrideFlagForModelMultiplyColors() and setOverrideFlagForModelScreenColors() to true, and then overwrite the member arrays with setMultiplyColor() and setScreenColor().
Tips
When the above process is performed, all subsequent Drawable colors will be set to the overwritten color information.
If you want to use the model’s original color settings again, you must add a separate process.