Multiply Color/Screen Color (Cocos Creator)
Updated: 03/14/2023
By applying Multiply Color and Screen Color to the model, tints can be changed in real time.
Multiply Color and Screen Color set in the Cubism Editor will be applied without any additional coding by using the SDK for Cocos Creator for Cubism 4.2 or later.
See the “Multiply Color/Screen Color” page in the Editor manual for details on setting the Multiply Color and Screen Color in the Cubism Editor.
In addition, by coding as needed, it is possible to manipulate Multiply Color and Screen Color from the SDK and perform the following operations.
- Apply Multiply Color and Screen Color interactively.
- Apply Multiply Color and Screen Color settings that have not been set in the Cubism Editor.
- Disable Multiply Color and Screen Color set in the Cubism Editor.
The following is an explanation of the procedure.
Procedures
The process is as follows.
- Model placement
- Overwrite flag settings for Multiply Color and Screen Color
- Multiply Color and Screen Color settings
Model placement
Place the model for which you want to set the Multiply Color and Screen Color in any scene.
Overwrite flag settings for Multiply Color and Screen Color
Set the overwrite flag for Multiply Color and Screen Color to true.
The default is false, which means that color information from the model is used.
There are two types of overwrite flags: one for the entire model, which is held by [CubismRenderController], and one for each Drawable object, which is held by [CubismRenderer].
Sample code is shown below.
It is assumed that the script is attached to the root object of the model as a component.
CubismRenderController renderController =Component.getComponent(CubismRenderController); renderController?.overwriteFlagForModelMultiplyColors = true; renderController?.overwriteFlagForModelScreenColors = true; renderController.renderers[0].overwriteFlagForDrawableMultiplyColors = true; renderController.renderers[0].overwriteFlagForDrawableScreenColor = true;
If the Overwrite flag for the entire model is enabled, it is possible to manipulate the Multiply Color and Screen Color from the SDK even if the Overwrite flag for an individual Drawable is disabled.
[CubismRenderController] is added as a component to the model root object and can be obtained by using getComponent.
[CubismRenderController] allocates an array of [CubismRenderer] for each Drawable object in the model, and each [CubismRenderer] can be referenced from [CubismRenderController].
Multiply Color and Screen Color settings
Define Multiply Color and Screen Color and set them to the model.
The code below shows the set values for setting red for the Multiply Color and green for the Screen Color for all Drawables.
The set color is stored in each [CubismRenderer] as a math.Color type.
In the example below, it is set in RGBA, but A is not used in the calculation of Multiply Color and Screen Color.
const multiplyColor = new math.Color(1.0, 0.5, 0.5, 1.0); const screenColor = new math.Color(0.0, 0.5, 0.0, 1.0); for (let i = 0; i < renderController.renderers.length; i++) { // MultiplyColor renderController.renderers[i].multiplyColor = multiplyColor; // ScreenColor renderController.renderers[i].screenColor = screenColor; }
Before applying Multiply Color and Screen Color:
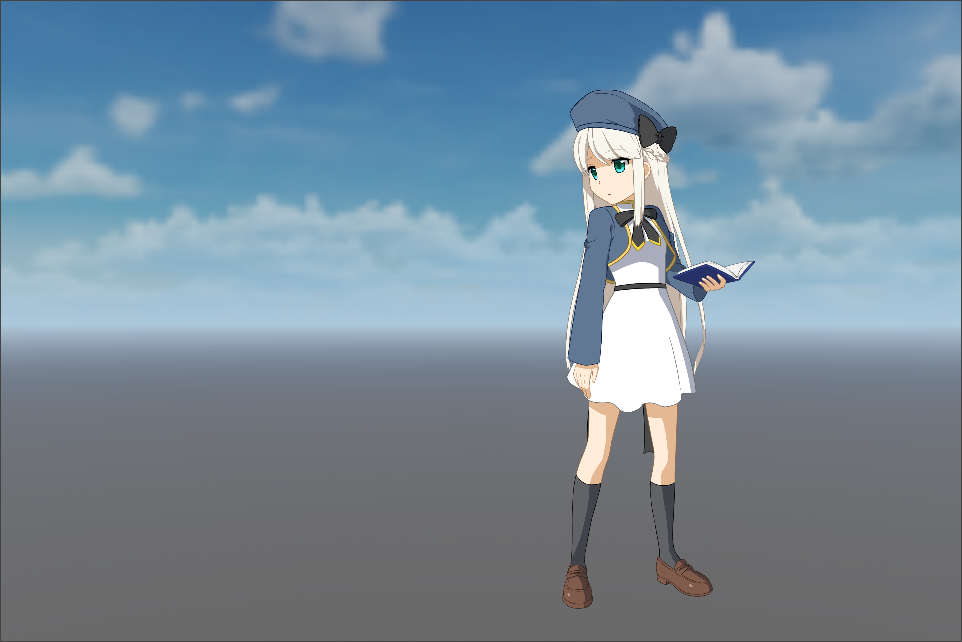
After applying red to Multiply Color and green to Screen Color:
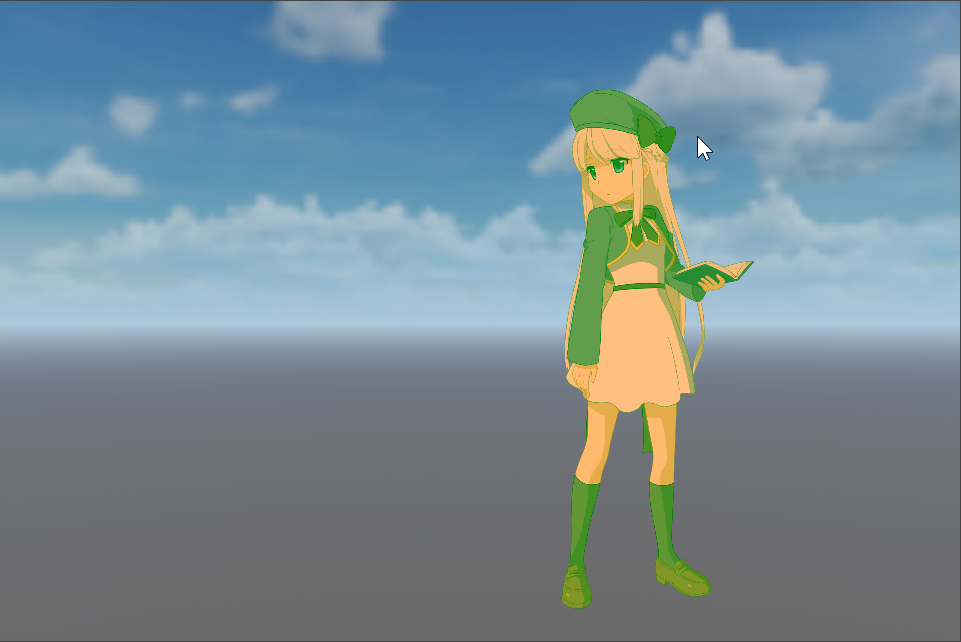
The model’s Multiply Color and Screen Color process is updated in all Drawables when there is a change in color information.
Tips
In this case, the same Multiply Color and Screen Color are set for all Drawables, but it is possible to set different Multiply Color and Screen Color for each Drawable.
To disable Multiply Color and Screen Color, set the following.
Multiply Color (1.0, 1.0, 1.0, 1.0)
Screen Color (0.0, 0.0, 0.0, 1.0)
Other Related Functions and Processes
Receive notification of Multiply Color and Screen Color updates from the model side
If a model parameter is associated with a change in Multiply Color/Screen Color, the model may change the Multiply Color/Screen Color when the model is animated, rather than from the SDK side.
The property isBlendColorDirty is implemented in [CubismDynamicDrawableData] to indicate that the Multiply Color and/or Screen Color have been changed.
This property is a flag that is set when either the Multiply Color or Screen Color is changed on the model side, and does not determine whether the Multiply Color or Screen Color is changed.
To handle [CubismDynamicDrawableData] data, the event OnDynamicDrawableData of [CubismModel] must be used.
Registering functions
let model: CubismModel; // Register listener. model.onDynamicDrawableData += onDynamicDrawableData;
Example of function to register
private onDynamicDrawableData(sender: CubismModel, data: CubismDynamicDrawableData[]): void { for (let i = 0; i < data.length; i++) { if (data[i].isBlendColorDirty) { console.log(i + ": Did Changed."); } } }