Raycast (UE)
Updated: 09/05/2024
This page contains statements regarding the alpha version.
Summary
Raycasting is a function that determines whether a user-specified Cubism mesh intersects with the set coordinates.
It is possible to obtain the clicked/tapped coordinates or a collision detection between any object in the scene and a specified mesh.
How to handle the component
Raycast in the Cubism SDK for Unreal Engine can be used by adding a CubismRaycastComponent to child components of a CubismModel actor.
If the ID of the ArtMesh to be raycasted is set in .model3.json, CubismRaycastComponent will be automatically added to the CubismModel actor as a child component during importing, so no configuration on the user side is required.
How to specify the target ArtMesh
If the ID of the ArtMesh to be raycasted is set in .model3.json, a CubismRaycastComponent will be automatically added as a child component to the CubismModel actor upon importing. If you want to manually add a parameter to be used for Raycast, select CubismRaycast from the “Details” tab, open “Live2D Cubism” -> “Parameters” from the list displayed at the bottom, and manually write the name of the parameter in the “Id” field of the index list that is created when a new index is inserted.
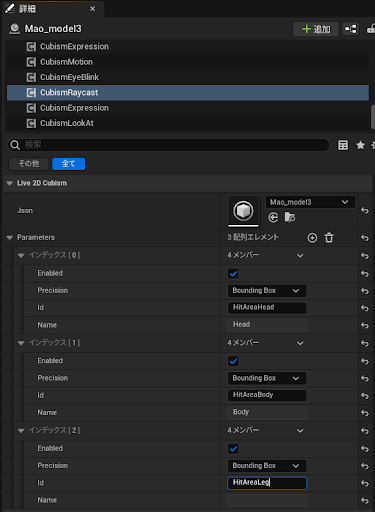
Applying parameters
CubismRaycastComponent performs individual intersection determination for each element of Parameters
. The ArtMeshes corresponding to the IDs set in Id
are the target of the intersection determination.
void UCubismRaycastComponent::Raycast( const FVector Origin, const FVector Direction, TArray<FCubismRaycastHit>& Result, const float Length ) const { Result.Empty(); for (const FCubismRaycastParameter& Parameter : Parameters) { if (!Parameter.bEnabled) { continue; } UCubismDrawableComponent* Drawable = Model->GetDrawable(Parameter.Id); if (!Drawable) { continue; } const FTransform& Transform = Drawable->GetComponentTransform(); FVector HitPosition, HitNormal; float HitTime; const FBox Box = Drawable->CalcBounds(Transform).GetBox(); const FVector NormDir = Direction.GetSafeNormal(); const FVector Start = Origin; const FVector End = Start + NormDir * Length; if (!FMath::LineExtentBoxIntersection( Box, Start, End, FVector::Zero(), HitPosition, HitNormal, HitTime )) { continue; } switch (Parameter.Precision) { case ECubismRaycastPrecision::BoundingBox: { // already checked break; } case ECubismRaycastPrecision::Mesh: { const TArray<int32> Indices = Drawable->GetVertexIndices(); TArray<FVector> Positions; for (const FVector2D& Position : Drawable->GetVertexPositions()) { Positions.Add(Model->GetRelativeTransform().TransformPosition(Drawable->ToGlobalPosition(Position))); } if (!RayMeshIntersection( Start, End, Positions, Indices, HitPosition, HitTime )) { continue; } break; } } FCubismRaycastHit RaycastHit; RaycastHit.Drawable = Drawable; RaycastHit.Distance = HitTime * Length; RaycastHit.GlobalPosition = HitPosition; RaycastHit.LocalPosition = Transform.InverseTransformPosition(HitPosition); Result.Add(RaycastHit); } }
To add or remove a target ArtMesh, add or remove the Parameters
element of the component.
When Enabled
is set to true, the ray intersection determination is performed.
The behavior of the intersection determination can be controlled by the parameters provided in CubismRaycastParameter.
- Precision
Specify the accuracy of the determination.
IfBoundingBox
is selected, the intersection determination is made for the AABB surrounding the ArtMesh, i.e., the cuboid along the axis. This results in a smaller computational load at the expense of lower precision in the intersection determination.
If this option is selected, it is recommended that the plane formed by the model be oriented in the same direction as one of the coordinate axes in order to optimize the AABB.
IfMesh
is selected, the intersection determination is accurate for the meshes that make up the ArtMesh. This results in a larger computational load at the expense of higher precision in the intersection determination.
Executing Raycast
The function provided in CubismRaycastComponent can be used to determine the intersection with the intersection determination object you have set up.
void Raycast( const FVector Origin, const FVector Direction, TArray<FCubismRaycastHit>& Result, const float Length = 10000.f ) const;
- Origin
Specify the position vector representing the origin of the ray to be flown. - Direction
Specify the direction vector of the ray to be flown. - Result
Specify an array to store the results of the intersection determination. - Length
Specify the length of the ray to be flown.