Load Models at Runtime
Updated: 01/30/2020
This section explains how to initialize and display the model from a script.
Summary
In Cubism 2.1, Init, loadModel, setTexture were used to initialize the model, load the model, and set the texture.
In Cubism 3.0 or later versions, LoadAtPath, ToModel can be used to initialize the model, load the model, and set the texture, as well as update and draw the model.
The first step is to create a script to load the model from the path.
The loaded model is then used ToModel() to display the model.
In this example, the script is written to load from StreamingAssets.
Details
Until Cubism 2.1, after initializing Live2D with Live2D.Init(), the model was loaded with Live2DUnity.loadModel.
Live2D.Init(); var live2dModel = Live2DModelUnity.loadModel((T)Resources.Load(path) as T);
The texture was then set as follows, and the model was updated and drawn with ALive2DModel.update() and ALive2DModel.draw().
live2dModel.setTexture(textureNumber, texture);
In Cubism 3.0 or later versions, the process has been completely redesigned. After loading the model using CusbimModel3Json.LoadAtPath, call .ToModel () to display the model.
Prefab is also created and displayed, and drawing is updated without writing .update() or .draw() update drawing from the script.
Now let’s initialize and display the model using the Cubism 3 SDK for Unity.
First, create a script to load the model from a path.
Create a new C# script and name it “InitModel.cs.”
Write InitModel.cs as follows.
using System; using System.IO; using Live2D.Cubism.Framework.Json; using UnityEngine; /// <summary> /// Initialize model. /// </summary> public class InitModel : MonoBehaviour { void Start () { //Load model. var path = Application.streamingAssetsPath + "/koharu.model3.json"; var model3Json = CubismModel3Json.LoadAtPath(path, BuiltinLoadAssetAtPath); var model = model3Json.ToModel(); } /// <summary> /// Load asset. /// </summary> /// <param name="assetType">Asset type.</param> /// <param name="absolutePath">Path to asset.</param> /// <returns>The asset on succes; <see langword="null"> otherwise.</returns> public static object BuiltinLoadAssetAtPath(Type assetType, string absolutePath) { if (assetType == typeof(byte[])) { return File.ReadAllBytes(absolutePath); } else if(assetType == typeof(string)) { return File.ReadAllText(absolutePath); } else if (assetType == typeof(Texture2D)) { var texture = new Texture2D(1,1); texture.LoadImage(File.ReadAllBytes(absolutePath)); return texture; } throw new NotSupportedException(); } }
Right-click in the Hierarchy window and click “Create Empty” to create an empty GameObject.
Then, attach InitModel.cs to the GameObject you created.
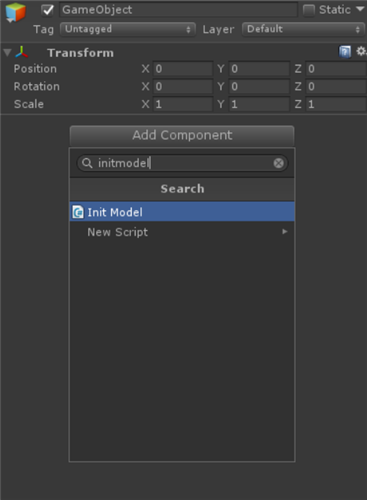
Next, add the model to the Assets folder for loading.
In this case, to load from StreamingAssets, select the Assets folder, right-click on the Project window, and create a new folder.
Rename the new folder “StreamingAssets.”
See Unity Manual: Streaming Assets for more information on StreamingAssets.
Add the model to StreamingAssets as shown in the image.
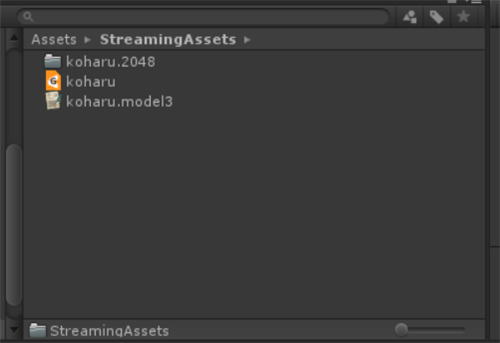
Lastly, press the Play button in Unity, and once the model is displayed, the operation is complete.
The model can now be initialized and displayed from a script.
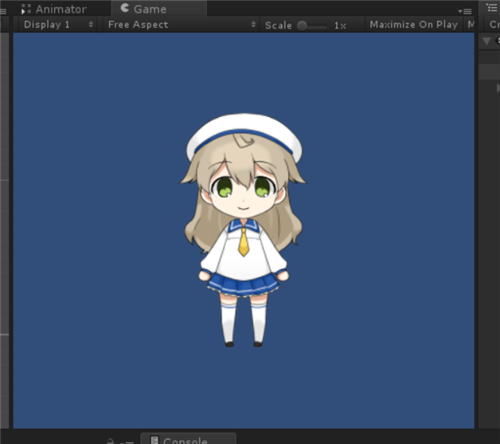
There is also an actual example using ToModel().
Please take a look at the following links as well.
[Live2D GitHub]CubismModel3Json.cs
https://github.com/Live2D/CubismUnityComponents/blob/develop/Assets/Live2D/Cubism/Editor/Importers/CubismModel3JsonImporter.cs
Here, the model’s GameObject is generated by ToModel on the hook of an import event in AssetImporter.
[Live2D GitHub]CubismViewer.cs
https://github.com/Live2D/CubismViewer/blob/develop/Assets/Live2D/Cubism/Viewer/CubismViewer.cs
Here, the model file is read from an absolute path, and then the model is generated with ToModel.
Original Workflow Method
[Added on 01/31/2019]
To make the Prefab created by .ToModel() in the Original Workflow method, pass true as an argument to CubismModel3Json.ToModel().
var model = model3Json.ToModel(true);