Customize Materials
Updated: 09/19/2017
by Traitam
Overview
This page first explains how Cubism models can be used in Unity and then, how to customize the material in the example using the model import function.
How Cubism Models Can Be Used in Unity
This section explains how the Cubism model is displayed in Unity Editor.
Summary
In Unity Editor, the material Live2D/Cubism/Materials/Unlit*.mat is usually used for display.
The material is applied to the model at the time of model import.
Details
When displaying Cubism models in Unity, the material Live2D/Cubism/Materials/Unlit*.mat is usually used.
This makes the display closer to how it is displayed in Cubism Editor.
The material is applied to the Cubism model when the model is imported into Unity Editor.
Therefore, the necessary materials for display are applied at the time the model is dragged and dropped into the Unity Editor.
When a Cubism model is imported into Unity, OnPickMaterial in CubismImporter.cs is called.
CubismBuiltinPickers.MaterialPicker is used here with the delegate.
With this method, a material is applied to each Drawable in the Cubism model.
It is then prefabricated and can be freely handled in Unity Editor.
If you want to customize a material from a script, OnPickMaterial is a delegate, so you can create a separate method and apply that method to OnPickMaterial to customize the material.
Next, customize the material of the specified Drawable from a script to display it, as shown in the following example.
Example: Customize the Material of the Specified Drawable
Change the material of a specified Drawable at the time of model import from a script.
Summary
Create the material you want to specify and reference the name of the specified Drawable.
For the specified Drawable, the specified material is used; otherwise, the normal material is used without customization.
Write a method to perform the above process and assign it to OnPickMaterial.
Details
The Drawable name specified in Unity Editor is the ID of the ArtMesh as set in Cubism Editor.
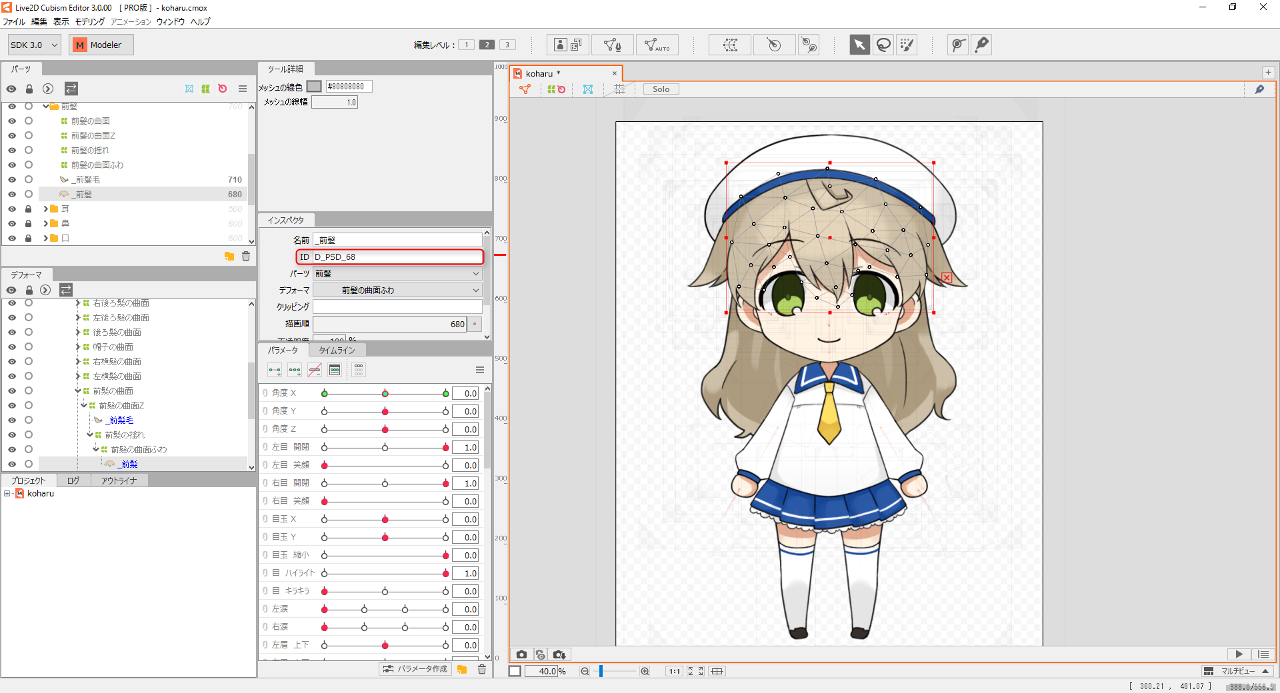
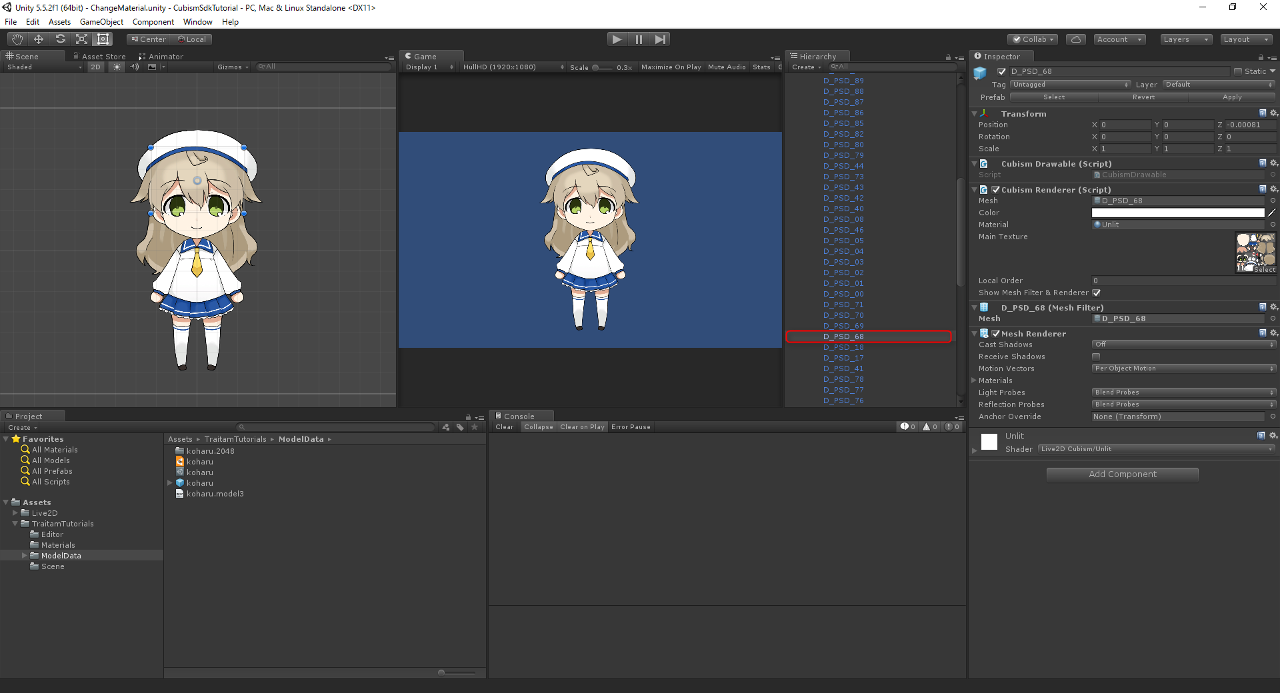
The Drawable name in Unity Editor is associated with the ArtMesh’s ID, which can be viewed in Cubism Editor, and cannot be changed in Unity Editor.
Therefore, to change the Drawable name, you must change the ID name in Cubism Editor.
In this example, to change the color of the entire hair, use hair Drawable.
When applying a material to the hair Drawable, apply a customized material.
Next, create a Material folder in Unity Editor and create a new material.
Name this material CustomMaterial. Change the shader of this material to Sprites/Default.
Here, change the color of the Tint to red.
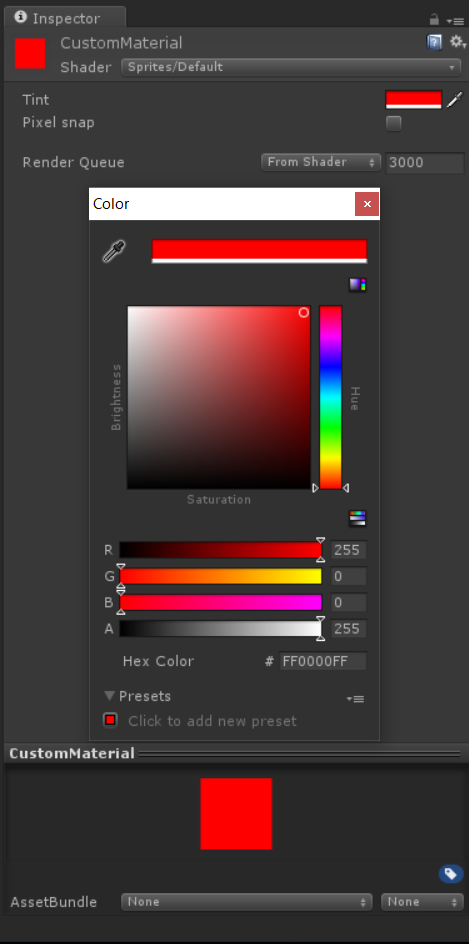
Finally, create an Editor folder and create a new C# script in that folder.
In this case, name it MaterialCustomizer.
In the script, refer to the specified Drawable and apply the material you just created if it is a Drawable of the same name.
Use normal materials (Live2D/Cubism/Materials/Unlit*.mat) for all other Drawables.
The code below includes that functionality.
using UnityEditor; using UnityEngine; using Live2D.Cubism.Core; using Live2D.Cubism.Framework.Json; using Live2D.Cubism.Editor.Importers; using System.Linq; namespace TraitamTutorials { /// <summary> /// Customizes materials of Cubism models from code. /// </summary> public static class MaterialCustomizer { /// <summary> /// IDs of drawables to customize. /// </summary> private static string[] _drawablesToCustomize = { "D_PSD_01" ,"D_PSD_02" ,"D_PSD_03" ,"D_PSD_04" ,"D_PSD_05" ,"D_PSD_68" ,"D_PSD_69" ,"D_PSD_70" ,"D_PSD_71"}; /// <summary> /// Custom material to assign. /// </summary> private static Material _customMaterial; /// <summary> /// Make custom material available. /// And select <see cref="Material"/> as custom material or model default material. /// </summary> [InitializeOnLoadMethod] private static void RegisterMaterialInitialize() { _customMaterial = AssetDatabase.LoadAssetAtPath<Material>("Assets/TraitamTutorials/Materials/CustomMaterial.mat"); CubismImporter.OnPickMaterial = CustomizeMaterial; } /// <summary> /// When <see cref="CubismDrawable.name"/> and <see cref="_drawablesToCustomize"/> the same, use custom material. /// Otherwise, use model default material. /// </summary> /// <param name="sender">Event source.</param> /// <param name="drawable">Drawable to pick for.</param> /// <returns><see cref="Material"/> for using the model.</returns> private static Material CustomizeMaterial(CubismModel3Json sender, CubismDrawable drawable) { //use custom material. if (_drawablesToCustomize.Any(n => n == drawable.Id)) { return _customMaterial; } //use default material. return CubismBuiltinPickers.MaterialPicker(sender, drawable); } } }
Now you can customize the material of the specified Drawable when importing the model.
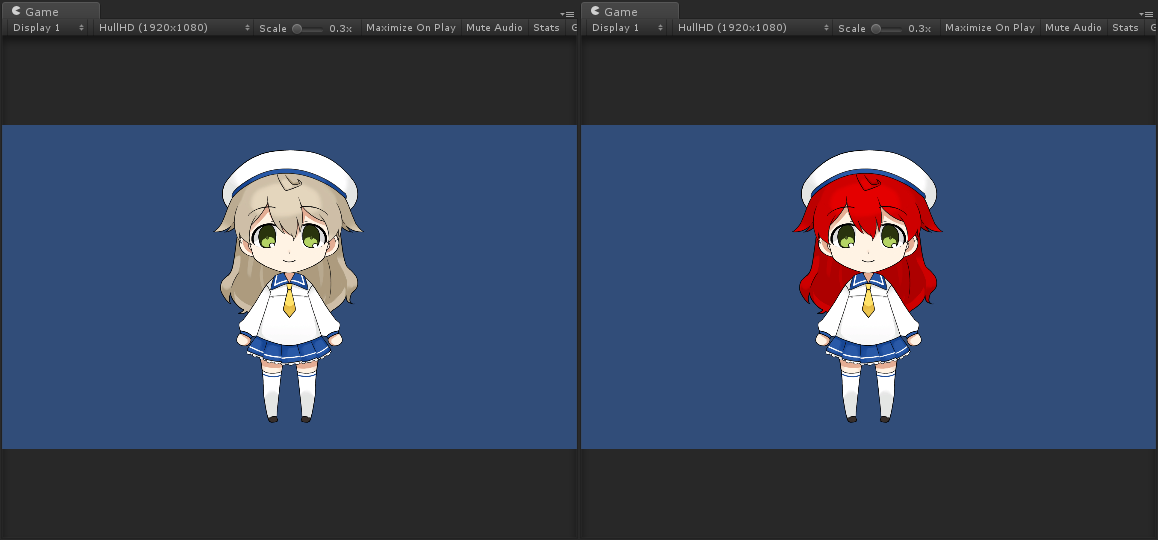