Collision Detection Settings (SDK for Cocos Creator)
Updated: 03/14/2023
This section describes how to obtain the model’s collision detection from the input coordinates.
The explanation is based on the assumption that the project is the same as the project for which the “Import SDK” was performed.
Summary
The Cubism SDK uses a component called Raycast for collision detection.
To set up Raycast, do the following three things.
- Attach Components to Perform Raycast
- Specify the ArtMesh to Be Used for the Collision Detection
- Get the Result of the Judgment from CubismRaycaster.raycast
Attach Components to Perform Raycast
Attach a component called [CubismRaycaster], which handles collision detection, to the Node that will be the root of the model.
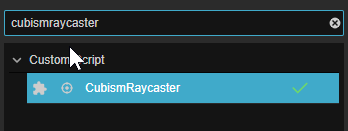
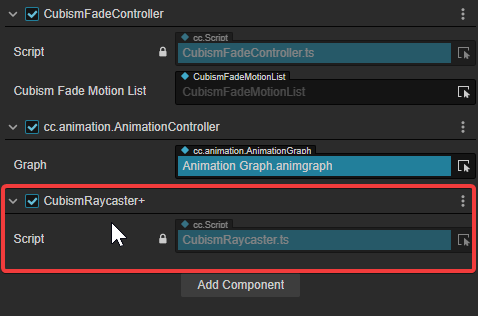
Specify the ArtMesh to Be Used for the Collision Detection
Nodes that manage each ArtMesh to be drawn are located under [Model]/Drawables/.
The name of the Node is the ID of its parameters.
From this Node, attach [CubismRaycastable] to the one that will be used as the range for the collision detection.
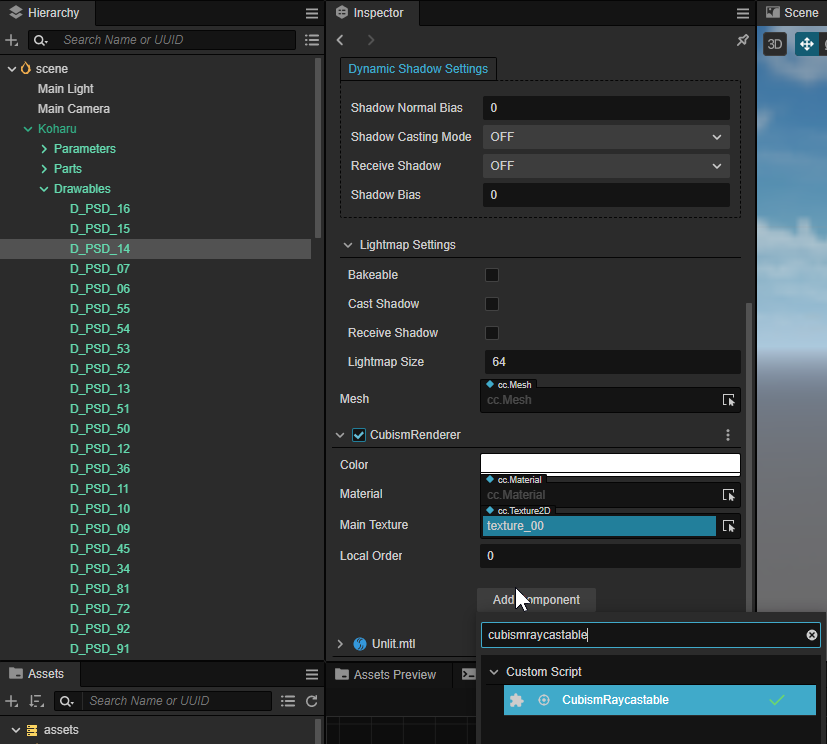
From CubismRaycastable, you can choose the range of collision detection for the attached mesh.
- Bounding Box: The rectangle surrounding the mesh is used for the collision detection. The load is lighter than with Triangles.
- Triangles: The shape of the mesh is used for the collision detection. If you want to determine the range accurately, please use this setting.
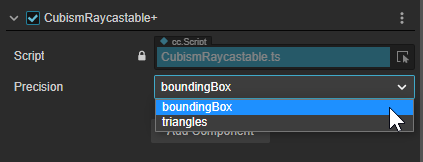
Get the Result of the Judgment from CubismRaycaster.raycast
Finally, use Raycast() on the CubismRaycaster attached to the root of the model to get the result of the collision detection.
Create a TypeScript script called “CubismHitTest,” add the code as follows, and attach it to the root of the model.
import { _decorator, Component, Node, input, Input, EventTouch, Camera } from 'cc'; import CubismRaycaster from '../../Framework/Raycasting/CubismRaycaster'; import CubismRaycastHit from '../../Framework/Raycasting/CubismRaycastHit'; const { ccclass, property } = _decorator; @ccclass('CubismHitTest') export class CubismHitTest extends Component { @property({ type: Camera, visible: true }) public _camera: Camera = new Camera; protected start() { input.on(Input.EventType.TOUCH_START,this.onTouchStart,this); } public onTouchStart(event: EventTouch) { const raycaster = this.getComponent(CubismRaycaster); if (raycaster == null) { return; } // Get up to 4 results of collision detection. const results = new Array<CubismRaycastHit>(4); // Cast ray from pointer position. const ray = this._camera.screenPointToRay(event.getLocationX(), event.getLocationY()); const hitCount = raycaster.raycast2(ray, results); // Show results. let resultsText = hitCount.toString(); for (var i = 0; i < hitCount; i++) { resultsText += "\n" + results[i].drawable?.name; } console.log(resultsText); } }
This completes the setup.
In this state, clicking on a mesh to which CubismRaycastable is attached in the Game View during execution will output the result of the collision detection to the Console View.
Example
D_PSD_16<CubismDrawable> D_PSD_15<CubismDrawable> D_PSD_14<CubismDrawable> D_PSD_07<CubismDrawable>