Update Model Parameters
Updated: 09/04/2019
This section is an explanation of the methods and notes on updating parameters attached to the model.
Summary
In Cubism 2.1 for Unity, it was necessary to update parameters by calling .update() after changing parameters with .setParamFloat(), etc.
In Cubism 3 and later versions of the Cubism SDK for Unity, updates are automatically performed from scripts attached to GameObjects generated by ToModel().
In addition, parameters can be updated from a separate script using parameter.value = value;.
Note that the parameters must be updated using LateUpdate() from Unity’s event function execution order.
The same care should also be taken when setting the order in which scripts are called in ScriptExecutionOrder.
Details
How to update with Cubism 2.1 SDK for Unity
In Cubism 2.1 SDK for Unity, updating parameters required calling the following method to perform the update.
void Update() { live2DModel.setParamFloat("Parameter ID", value); live2DModel.update(); }
In detail, the code was as follows.
void Update() { double timeSec = Time.time; double t = timeSec * 2 * Math.PI; // Breath live2DModel.setParamFloat(breath, (float)(0.5f + 0.5f * Math.Sin(t / 3.2345)), 1); // Update parameter values live2DModel.update(); }
How to update Cubism 3 or later versions with the Cubism SDK for Unity
In Cubism 3 and later versions of the Cubism SDK for Unity, parameters are automatically updated via CubismRenderController.cs attached to the generated Prefab.
This can be used to update the drawing by applying the values changed by the animation to the parameters.
To apply a value to a parameter, use the following.
void LateUpdate() { parameter = model.Parameters[index]; parameter.Value = value; }
Examples of specific descriptions are as follows.
using UnityEngine; using Live2D.Cubism.Core; using Live2D.Cubism.Framework; public class ParameterLateUpdate : MonoBehaviour { private CubismModel _model; private float _t; private void Start() { _model = this.FindCubismModel(); } private void LateUpdate() { _t += (Time.deltaTime * 4f); var value = Mathf.Sin(_t) * 30f; var parameter = _model.Parameters[2]; parameter.Value = value; } }
This is the code used in the GIF described below.
model.Parameters[index] is set to “2” because the parameter PARAM_ANGLE_Z of koharu is the second (starting from 0).
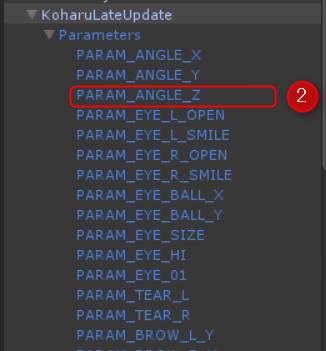
Note that LateUpdate() must be used when updating parameters from a script using Cubism 3 or later of Cubism SDK for Unity.
Unity animations are processed after Update(). Therefore, changing the value of a parameter within Update() will overwrite the animation.
To work around this, the parameters must be updated using LateUpdate(), which is called after the animation process is complete.
The following GIF shows a Scene in which the PARAM_ANGLE_Z value is manipulated from a script on a model playing an animation.
The left model manipulates parameter values from Update() and the right from LateUpdate(), and you can see that the left one has had its value changes overwritten.
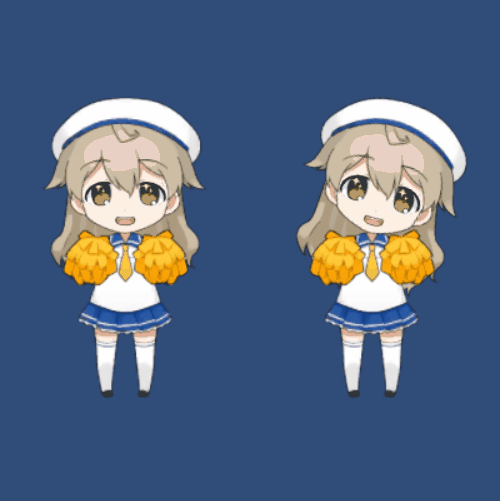
Tips1
For the GIF shown above as an example, this is set up by overriding the animation parameters. Therefore, parameters that are being updated from scripts have no animation movement applied to them.
If you want to use the parameters in conjunction with animation, you need to change the Blend Mode with CubismParameterBlendMode, which is included in Live2D.Cubism.Framework. There are three Blend Modes.
- Override…overwrite and update parameters
- Additive…add and update parameters
- Multiply…multiply and update parameters
We encourage you to use the Blend Mode that best suits your application.
The following is sample code.
Tips2
Although model parameters can be obtained using the method described for updating with the Cubism 3 or later version of Cubism SDK for Unity, certain parameters can also be obtained using the following methods.
This is the code to get ParameterID by using FindById() included in Live2D.Cubism.Core.
By attaching a script with this code to the root of the model, the specified parameter (ParameterID) can be obtained.
Tips3
Other points to note are that you should also be careful if you are changing the order in which scripts are executed in ScriptExecutionOrder.
Depending on the order in which the scripts are executed, the timing of parameter updates may change and parameters may not update properly.