Play back motion from a script
Updated: 01/30/2020
This page describes the procedure for setting up MotionController for Cubism models.
The following explanation is based on the assumption that the project is the same as the project for which the “Import SDK” was performed.
Summary
If you want to play animations from scripts without using Unity’s Mecanim, the Cubism SDK for Unity allows you to set this up by using a component called “CubismMotionController.”
See “Motion” for more information on CubismMotionContoller.
CubismMotionController requires the Animator component to operate.
However, CubismMotionController cannot coexist with motion playback using Animator’s AnimatorController.
Therefore, when using CubismMotionController to play motion, do not set AnimatorController to Animator.
To make the above settings for a Cubism model, follow the steps below.
- Attach CubismMotionController
- Prepare a component that calls the instruction to play the AnimationClip
- Create a button to play the motion
1. Attach CubismMotionController
Attach “CubismMotionController” to the GameObject that will be the root of the model to play the animation.
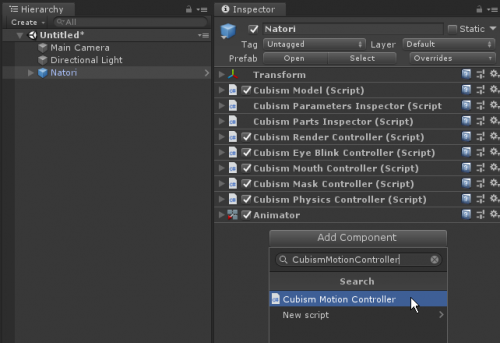
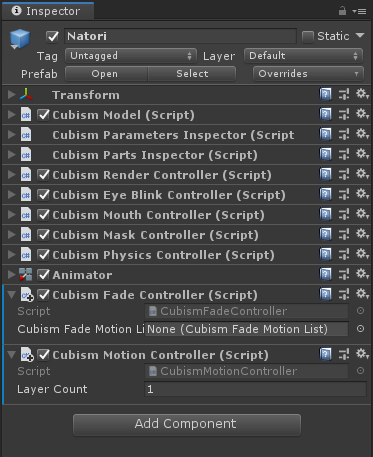
CubismMotionController has one setting item.
– Layer Count: Total number of animation layers managed by CubismMotionController. The range of values to be set is 1 or more.
When a CubismMotionController is attached to a GameObject, if neither Animator nor CubismFadeController is attached to that GameObject, they will be attached at the same time.
CubismMotionController requires Animator because it uses Playable for motion playback.
If the AnimatorController is set as the Controller of the Animator component, it will take precedence.
If you are using CubismMotionController, do not set anything here.
CubismFadeController is also required because CubismMotionController uses MotionFade to blend one motion with another motion.
If CubismFadeController.CubismFadeMotionList is not set, set the generated .fadeMotionList.
See “Enable Motion Fade in Mecanim” for more information on .fadeMotionList settings.
2. Prepare a Component that Calls the Instruction to Play the AnimationClip
Create a C# script called “MotionPlayer” and rewrite the code as follows.
Here, the argument AnimationClip is made to play.
using Live2D.Cubism.Framework.Motion; using UnityEngine; public class MotionPlayer : MonoBehaviour { CubismMotionController _motionController; private void Start() { _motionController = GetComponent<CubismMotionController>(); } public void PlayMotion(AnimationClip animation) { if ((_motionController == null) || (animation == null)) { return; } _motionController.PlayAnimation(animation, isLoop: false); } }
Attach the MotionPlayer you created to the GameObject to which you attached the CubismMotionController in Step 1.
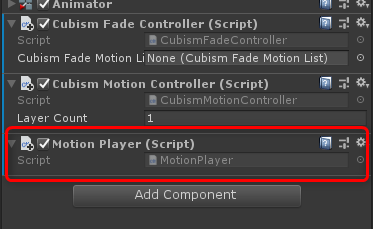
This completes the setup for playing back motion from a script.
You can play the motion by calling MotionPlayer.PlayMotion() from an external script etc. and passing the AnimationClip you want to play as an argument.
Here, call this function from a button.
3. Create a Button to Play the Motion
Create a Button in the Scene by clicking on the Create button at the top of the Hierarchy window or by right-clicking on the Hierarchy window and clicking on UI > Button.
The created Button can be placed anywhere.
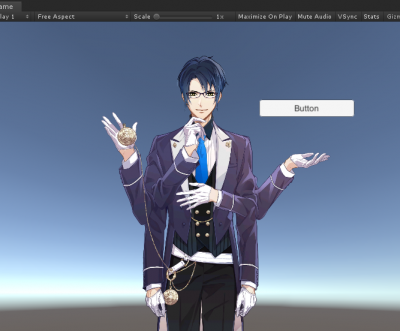
Select the Button you created and click the “+” at the bottom of the Button’s “On Click()” from the Inspector window.
Drag and drop the GameObject of the model in the Heirarchy onto the added list and select MotionPlayer / PlayMotion() from the drop-down menu on the right.
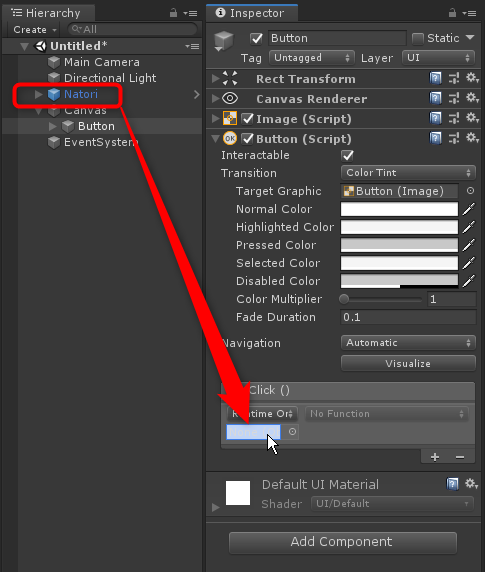
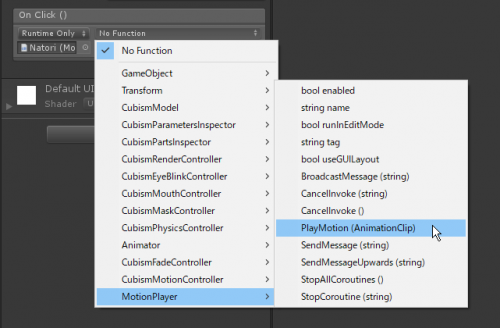
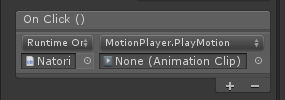
Finally, set the AnimationClip to be played as the argument.
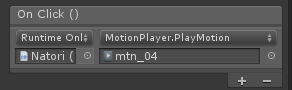
This completes the setup. When executed in this state, the motion will play when the button in the Game View is clicked.
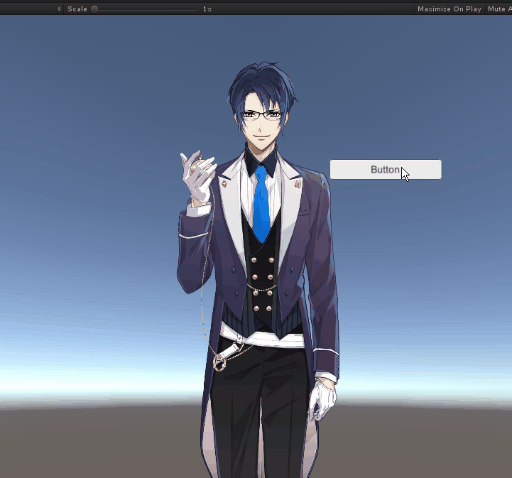