Control the Order of Execution of Your Own Components
Updated: 01/22/2020
This section describes the procedure for controlling the order of execution among other Cubism components for a user’s own component.
The following explanation is based on the assumption that the project is the same as the project for which the [Import SDK – Place Models] was performed.
Summary
Some components in the Original Workflow of the Cubism SDK for Unity have restrictions on the order in which they are executed.
In the Cubism SDK for Unity, this can be controlled by using CubismUpdateController, which controls the order of execution of the above components.
The component controlled by CubismUpdateController will be the component attached to the root of the Prefab of the Cubism model.
With CubismUpdateController, the user’s own components can be controlled in the same order of execution.
As an example, this section describes the procedure for setting up execution order control for the following components.
public class CubismExampleController : MonoBehaviour { private void Start() { // Initialization process of CubismExampleController } private void LateUpdate() { // Update process of CubismExampleController } }
1. Attach Components to Prefab
Attach CubismExampleController to the GameObject at the root of the Prefab placed in the Hierarchy.
If Prefab is not imported in OW format, CubismUpdateController is also attached.
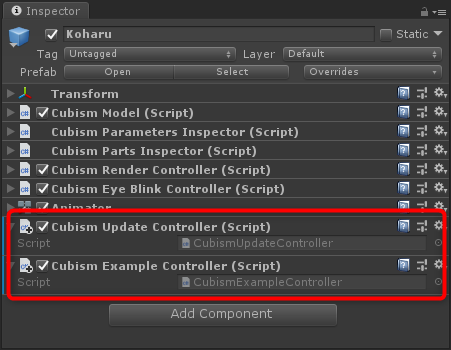
2. Implement ICubismUpdatable in the Component
Implement the ICubismUpdatable interface in the component that controls the execution order.
CubismUpdateController retrieves components that implement ICubismUpdatable at runtime and controls the order in which they are executed.
public class CubismExampleController : MonoBehaviour, ICubismUpdatable { // Whether the order of execution is controlled during non-execution of a Scene public bool NeedsUpdateOnEditing { get { return false; } } // Execution order of this component public int ExecutionOrder { get { return 0; } } // Update functions which are controlled by the order of execution public void OnLateUpdate() { } // Whether the order of execution is controlled public bool HasUpdateController { get; set; } private void Start() { // Initialization process of CubismExampleController } private void LateUpdate() { // Update process of CubismExampleController } }
The ICubismUpdatable interface implemented here is as follows.
namespace Live2D.Cubism.Framework { /// <summary> /// Cubism update interface. /// </summary> public interface ICubismUpdatable { int ExecutionOrder { get; } bool NeedsUpdateOnEditing { get; } bool HasUpdateController { get; set; } void OnLateUpdate(); } }
ExecutionOrder is the value that determines the order of execution for this component.
The smaller this value, the earlier it is called in relation to other components.
The values set for the components included with the SDK are described in the CubismUpdateExecutionOrder.
HasUpdateController is a flag that allows components implementing ICubismUpdatable to be called from Unity event functions if the CubismUpdateController is not attached.
public static class CubismUpdateExecutionOrder { public static readonly int CubismFadeController = 100; public static readonly int CubismPoseController = 200; public static readonly int CubismExpressionController = 300; public static readonly int CubismEyeBlinkController = 400; public static readonly int CubismMouthController = 500; public static readonly int CubismHarmonicMotionController = 600; public static readonly int CubismLookController = 700; public static readonly int CubismPhysicsController = 800; public static readonly int CubismRenderController = 10000; public static readonly int CubismMaskController = 10100;
3. Make Components Compatible with CubismUpdateController
Modify CubismExampleController as follows.
public class CubismExampleController : MonoBehaviour, ICubismUpdatable { // Whether the order of execution is controlled during non-execution of a Scene public bool NeedsUpdateOnEditing { get { return false; } } // Execution order of this component public int ExecutionOrder { get { return 150; } } // Update functions which are controlled by the order of execution public void OnLateUpdate() { // Update process of CubismExampleController } // Whether the order of execution is controlled public bool HasUpdateController { get; set; } private void Start() { // Initialization process of CubismExampleController // Check if CubismUpdateController is attached to the model’s Prefab HasUpdateController = (GetComponent<CubismUpdateController>() ! = null); } private void LateUpdate() { // If CubismUpdateController is not attached, update process is performed from CubismExampleController’s own event function if (!HasUpdateController) { OnLateUpdate(); } } }
The update process performed by LateUpdate() is moved to OnLateUpdate(), which is called by CubismUpdateController.
This completes the setup for controlling the order of execution.
When this script is attached to the Prefab of the Cubism model and the scene is executed, the update process of this script is called by the CubismUpdateController.